In the realm of server-side JavaScript, Node.js stands as a beacon of innovation, enabling developers to craft sophisticated, high-performance applications across diverse domains.
However, harnessing the full potential of Node.js requires more than just basic knowledge of its syntax and core concepts. To truly excel in Node.js development and create robust, maintainable applications, it’s essential to adopt a set of best practices that leverage the strengths of the platform while mitigating common pitfalls.
Whether you’re a seasoned Node.js developer looking to refine your skills or a newcomer eager to learn the ropes, this blog series will provide invaluable insights, tips, and strategies to help you write cleaner code, optimize performance, and build resilient applications.
From project structure and modularization to error handling, security, and deployment strategies, we’ll explore a wide range of topics essential for mastering Node.js development.
Let’s dive in and discover the path to Node.js mastery together!
In this series, we will explore eight crucial software development aspects: project architecture, error handling, code style, testing, quality assurance, preparing for production deployment, security measures, performance optimization, and Docker usage.
Each article will provide concise insights and best practices for mastering the Node.js elements of software engineering.
- Part-1: Project Architecture Practices
- Part-2: Error Handling Practices
- Part-3: Code Style Practices
- Part-4: Testing And Quality Practices
- Part-5: Ready To Production Practices
- Part-6: Security Practices
- Part-7: Performance Practices
- Part-8: Docker Practices
Part-1: Project Architecture Practices
In this article, our focus will be on project architecture and best practices.
1.1 Organize Your Solution Around Distinct Business Components:
Having a good starting point when it comes to our project architecture is crucial for the longevity of the project itself and for effectively addressing future changing needs.
At the heart of the system’s structure should lie folders or repositories, each representing appropriately sized business modules. These modules encapsulate distinct product domains, embodying their own API, logic, and dedicated database. It helps ease development hurdles and alleviates deployment anxieties.
Consequently, developers gain the ability to iterate at a swifter pace. Achieving this doesn’t mandate physical segregation; it can be accomplished through either a Monorepo or a multi-repo approach.
Here is an example of a good folder structure where structure is designed by self-contained components.
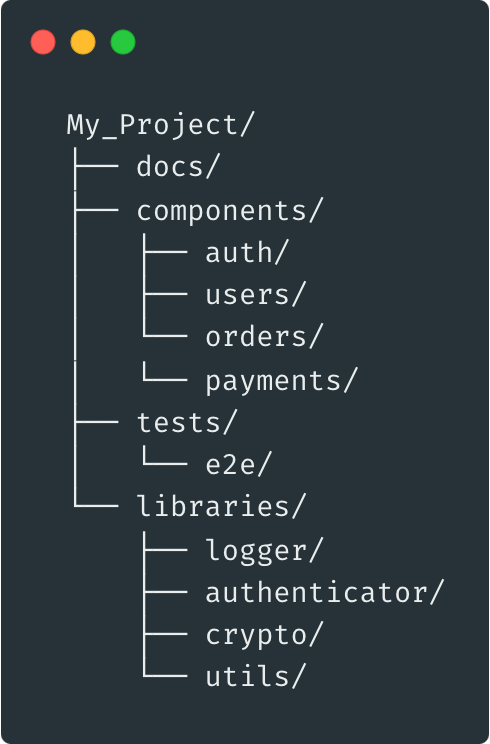
Here is an example of bad folder structure.
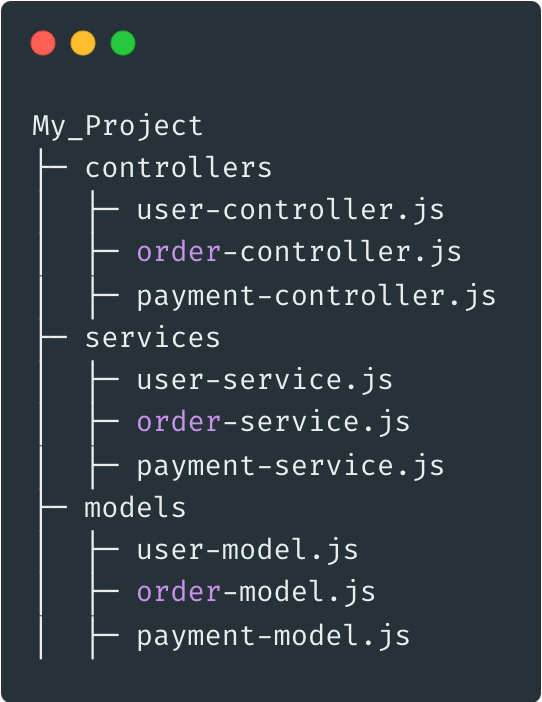
1.2 Layer Your Components Into Three-Tiered:
The root of every component should hold 3 folders that represent common concerns and stages of every transaction:
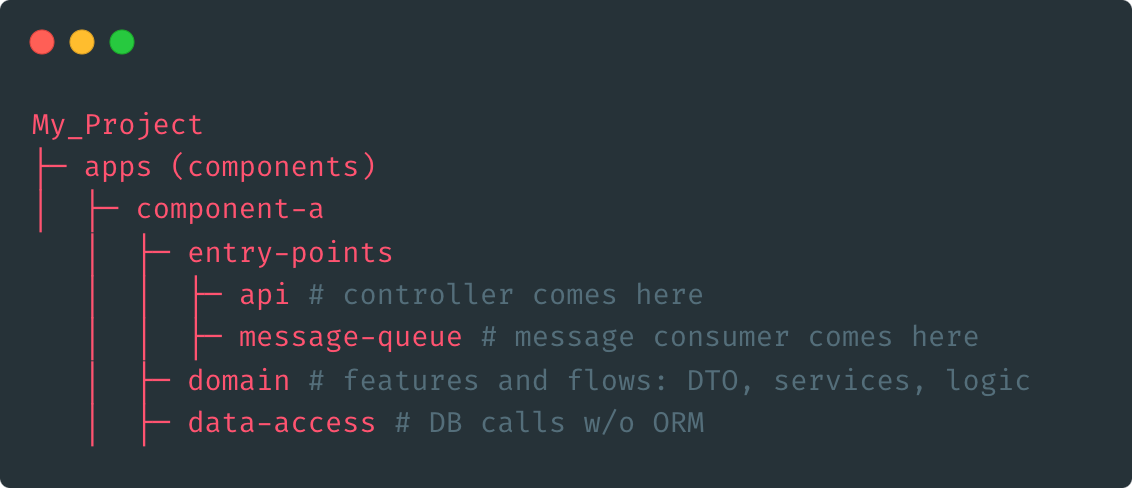
Entry-points
At the application’s entry point, whether through REST APIs, Graph APIs, message queues, or scheduled jobs, lies a pivotal layer responsible for adapting incoming payloads to the application’s format.
This adapter, often termed as a “controller,” performs minimal tasks including preliminary validation, invocation of the logic/domain layer, and generating a response.
Despite its brevity, this layer plays a crucial role in orchestrating seamless communication between external interfaces and the core functionality of the application.
Domain
Within this layer resides the core essence of the application, where the flow of operations, logical processes, and data management converge.
Here, the system accommodates protocol-agnostic payloads in the form of plain JavaScript objects and likewise produces such objects as output.
Data-access
This is the section of the application dedicated to managing interactions with the database.This layer involves DB helper utilities like query builders, ORMs, DB drivers and other implementation libraries. This layer is also designed to handle the exchange of data.
In agile infrastructures, developers zoom in on the domain folder for rapid feature deployment, reducing technical fuss. This core strategy echoes across architectures like DDD, hexagonal, and clean architecture, turbocharging value-driven tasks. Moreover, keeping domain layers protocol-agnostic boosts versatility, extending services to diverse client needs beyond HTTP.
If simplicity and clarity are desired in the code, MVC may be best; whereas if flexibility and modularity are desired in the code, clean architecture may be best.
1.3 Package Common Utilities:
Place all reusable modules in a dedicated folder, e.g., “libraries”, and underneath each module in its own folder, e.g., “/libraries/logger”.
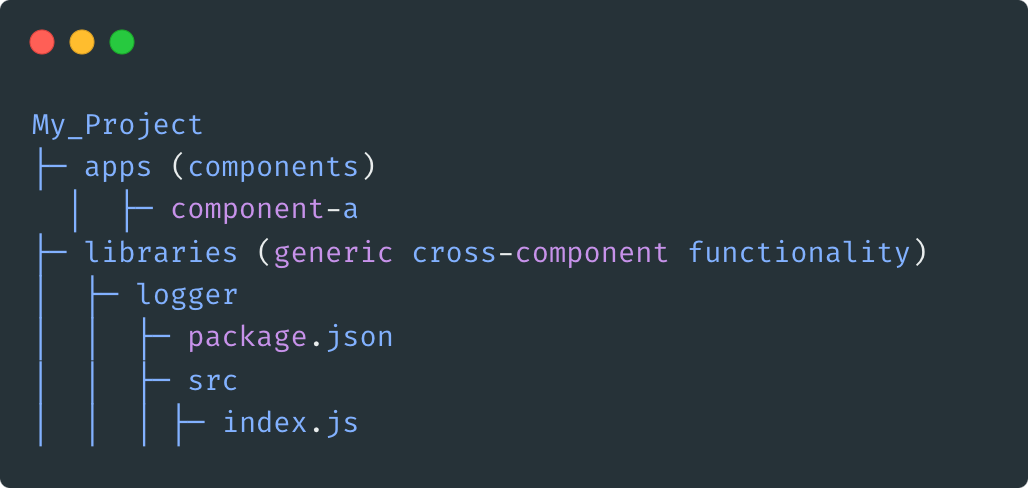
Modularizing utilities as npm packages promotes code organization and scalability, allowing teams to efficiently manage and update shared functionalities across multiple projects.
This approach facilitates code sharing, reduces redundancy, and accelerates development cycles by enabling developers to leverage pre-built solutions rather than reinventing the wheel.
In summary, wrapping common utilities as npm packages empowers developers with a robust ecosystem of reusable components, fostering efficiency, consistency, and collaboration in software development workflows.
1.4 Secure and Hierarchical Configuration Management:
- Tedious Environment Variable setup: Manually setting up numerous keys using process environment variables can be laborious, especially with a large number of configurations. However, relying solely on configuration files can limit flexibility. To strike a balance, a robust solution combines configuration files with the ability to override settings using process variables, providing both ease of use and flexibility for DevOps administrators.
- Flat JSON Structures: Managing configurations in flat JSON files can quickly become unwieldy as the list of keys grows. Organizing configurations into hierarchical structures grouped into sections can greatly improve readability and maintainability. Some configuration libraries offer the capability to merge settings from multiple files at runtime, simplifying management further.
- Handling Sensitive Information: Storing sensitive information like database passwords securely is paramount. While conventional methods may include encrypting files or omitting actual values and relying on environment variables during deployment, no one-size-fits-all solution exists. Developers must carefully evaluate the trade-offs and select the most appropriate approach based on their security requirements and deployment environment.
- Advanced Configuration Scenarios: In certain scenarios, injecting configuration values via command-line arguments or synchronizing settings across multiple servers using a centralized cache like Redis is necessary. Implementing these advanced features requires a flexible configuration solution that can accommodate diverse deployment architectures and scaling requirements.
- Fail-Fast Mechanisms: Ensuring that the application fails quickly and provides immediate feedback in case of missing or invalid configuration variables is crucial for maintaining system integrity. Tools like Convict can help validate configurations during startup, minimizing the risk of runtime errors caused by misconfigurations.
1.5 Choosing the Right Framework:
When building apps and APIs, picking the right framework is crucial. With so many options out there, it’s easy to miss some good ones or forget important things, ending up with a not-so-great choice.
Let’s talk about a few Node.js frameworks that are worth considering: Nest Js, Fastify, express, and Koa.
Choosing the main framework is a big deal because it affects how smoothly your development goes and how likely you are to run into problems.
We think it’s smart to go for the most popular frameworks—the ones with the most downloads and stars on GitHub. So, here’s a rundown of the good and not-so-good parts of each framework, and some tips on which one might be best for your project.
Express.js
Pros: Unmatched popularity; gigantic eco-system of extensions and middleware; simple to learn and use; familiar to almost every Node.js developer; tons of community articles and videos are based on express
Cons: Covers a small subset of a typical application needs – merely a web server that invokes the app function per URL. Choosing express means leaving a handful of app concerns uncovered; outdated mechanics – no native support for async-await; barely maintained and updated; Slower than others.
Nest.js
Pros: More batteries than any other option – covers many application concern including message queues, scheduled jobs and more; OOP-style is an advantage for teams who appreciate this design style; awesome docs; well-maintained; high popularity with a vibrant community
Cons: Cloud-native Node.js conventions provide high-level abstractions. However, teams may face heightened complexity due to the incorporation of numerous features, extensive TypeScript usage, and reference to advanced patterns. Additionally, the presence of several unique concepts like interceptors, guards, and modules contributes to a steeper learning curve. Overall, the framework is highly opinionated.
Fastify
Pros: Relatively simple and lean; mostly based on Node.js/JavaScript standards; relatively shallow learning curve; with its official plugins cover many application concerns though not as rich as Nest Js.
Cons: Younger than others and not as popular yet; smaller ecosystem compared to express and Nest Js.
Koa
Pros: When compared with express: it’s Simpler and nimbler; modern API with async/await support; better performance.
Cons: Covers a small subset of a typical application needs – leaves a handful of app concerns uncovered; Not as popular as express and Nest Js.
Additional framework choosing guide.
Prefer express js when – have an experienced architect available and require meticulous control over the finer aspects of your project. Alternatively, consider Koa, which offers a modern API compared to Express, albeit with a smaller ecosystem.
Prefer fastify when – The application comprises moderately sized components or microservices, rather than a large monolithic structure, and is best suited for teams with strong expertise in JavaScript and Node.js. Maintaining alignment with the ethos and principles of Node.js is preferred in this scenario.
Prefer Nest Js when – need to design and code in OOP style; when the team is highly experienced with Java/Spring/Angular or similar; for large size app that can’t be broken down (i.e. monolith) to autonomous component; when the decision-making overhead should be minimized; when the time to the first delivery is a critical factor.
1.6 Provide Type Safety:
Coding without type safety is no longer an option, TypeScript is the most popular option for this mission. Use it to define variables and functions return types. Researches show that using TypeScript can help in detecting ~20% of bugs earlier.
TypeScript has garnered widespread acceptance within the community and is nearly regarded as a standard for contemporary JavaScript applications.
It significantly enhances coding ergonomics compared to plain JavaScript, facilitating improved editor code completions even for legacy libraries written in JavaScript, and has demonstrated efficacy in preventing certain types of bugs.
However, upon closer examination, TypeScript presents two distinct offerings: type-safety and advanced design constructs such as abstract classes, interfaces, namespaces, and more.
Final Words
In conclusion, adopting effective project architecture practices lays the foundation for robust, scalable, and maintainable Node.js applications.
Stay tuned for more insights on mastering Node.js through best practices in the upcoming parts of this series.